Where authentication is supplied via HTTP headers and payload.json is a JSON object:
INTRO
Getting Started Authentication SDKsHLR LOOKUPS
POST
/hlr-lookup
POST
/hlr-lookups
POST
/hlr-lookups/url
NT LOOKUPS
POST
/nt-lookup
POST
/nt-lookups
POST
/nt-lookups/url
MNP LOOKUPS
POST
/mnp-lookup
GET
/mnp-coverage
MCCMNCs
GET
/mccmncs
ACCOUNT
GET
/balance
HELPER ENDPOINTS
GET
/ping
GET
/time
GET
/auth-test
LEGACY API
Legacy API DocsGetting Started
All mobile network operators are connected within a system called the SS7 signaling network. The SS7 transports data about subscribers, networking, voice calls, and SMS text messages between the carriers. Real-time context and status information about every mobile phone is stored in databases called Home Location Registers, which form the backbone of subscriber information for a mobile network.
HLR Lookups is a technology to query home location registers and acquire live networking and connectivity information about mobile phone numbers, such as, whether a mobile phone is switched on or switched off, to which network it belongs, whether it is currently roaming, if a number has been ported between networks, and if it is valid or invalid.
The HLR Lookups API supplies businesses with real time queries towards the home location registers of all mobile networks. This documentation helps you to implement HLR Lookups into your own software to automatically receive real time mobile phone information when needed.
Using the HLR Lookups API
Performing HLR Lookup queries is fast, secure, and easy. After you've signed up and obtained your API Key, all you need to do is create HTTP POST requests for your target mobile phone numbers.
Example Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/hlr-lookup' \
-H "X-Digest-Key: YOUR_API_KEY" \
-H "X-Digest-Signature: DIGEST_AUTH_SIGNATURE" \
-H "X-Digest-Timestamp: UNIX_TIMESTAMP" \
-d "@payload.json"
Example Payload
{
"msisdn":"+14156226819"
}
In response you receive real-time mobile phone number status information from mobile network operators directly.
Success Response application/json
{
"id":"f94ef092cb53",
"msisdn":"+14156226819",
"connectivity_status":"CONNECTED",
"mccmnc":"310260",
"mcc":"310",
"mnc":"260",
"imsi":"***************",
"msin":"**********",
"msc":"************",
"original_network_name":"Verizon Wireless",
"original_country_name":"United States",
"original_country_code":"US",
"original_country_prefix":"+1",
"is_ported":true,
"ported_network_name":"T-Mobile US",
"ported_country_name":"United States",
"ported_country_code":"US",
"ported_country_prefix":"+1",
"is_roaming":false,
"roaming_network_name":null,
"roaming_country_name":null,
"roaming_country_code":null,
"roaming_country_prefix":null,
"cost":"0.0100",
"timestamp":"2020-08-07 19:16:17.676+0300",
"storage":"SYNC-API-2020-08",
"route":"IP1",
"processing_status":"COMPLETED",
"error_code":null,
"data_source":"LIVE_HLR"
}
Please continue to POST /hlr-lookup
for a detailed documentation of the HTTP response attributes and mobile phone connectivity statuses.
Mobile Number Portability APIs
Mobile number portability (MNP) lookups reliably determine portability and network information (but do not indicate whether the target mobile phone is currently connected to a network and reachable). Inspect the POST /mnp-lookup
endpoint If your primary use-case is the extraction of the MCCMNC of a mobile phone number and live connectivity status is irrelevant to you.
Number Type Detection APIs
The POST /nt-lookup
lookup endpoint helps you reliably determine whether a given phone number belongs to landline, mobile, premium rate, VoIP, pager, or other numbering plan ranges in real-time. Unlike HLR and MNP queries it does not take portability into account.
Software Development Kits (SDKs)
The HLR Lookups API works with any REST client in any programming language and we've published SDKs for PHP, Ruby, and NodeJS on our GitHub to help you get started quickly.
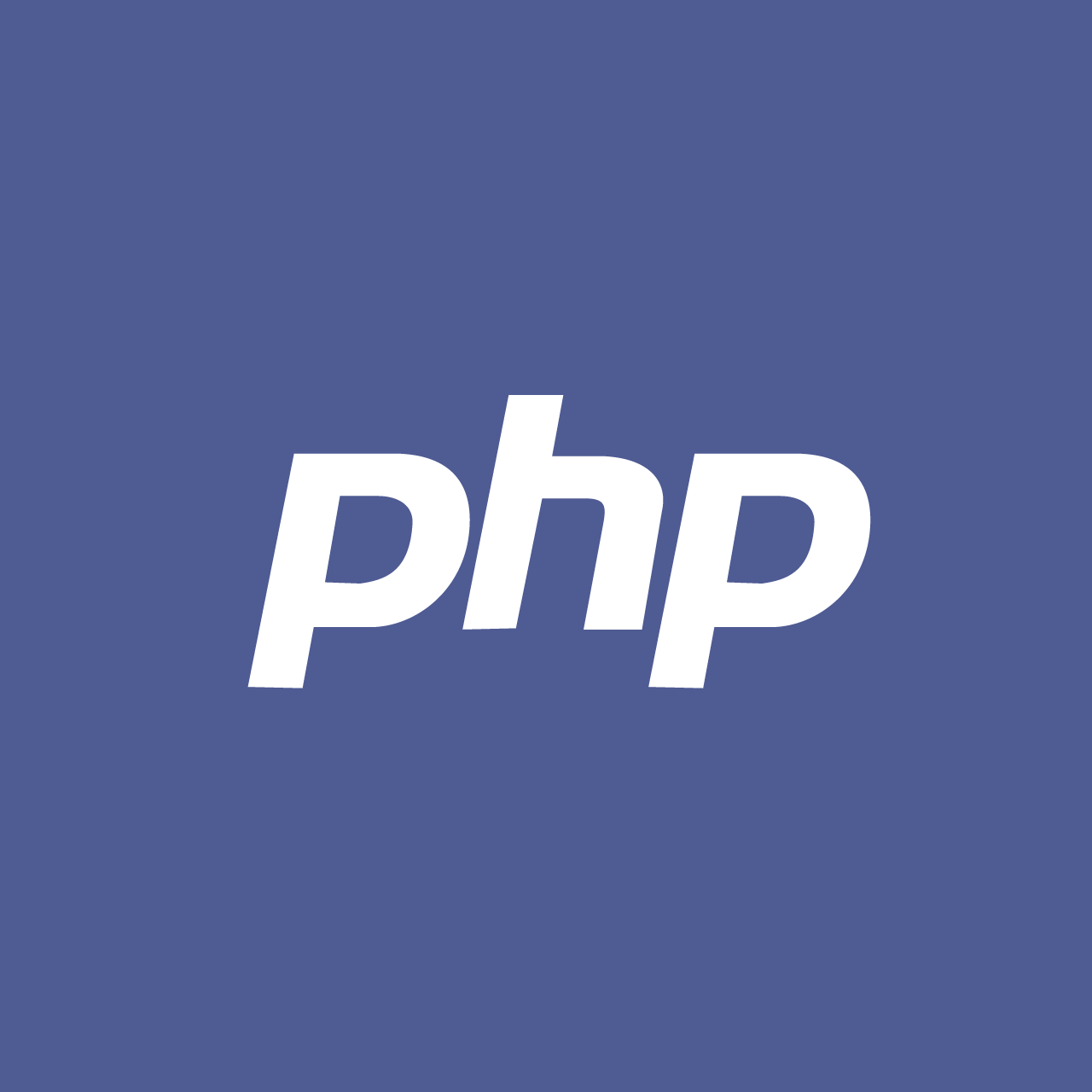
PHP
Official HLR Lookup SDK for PHP
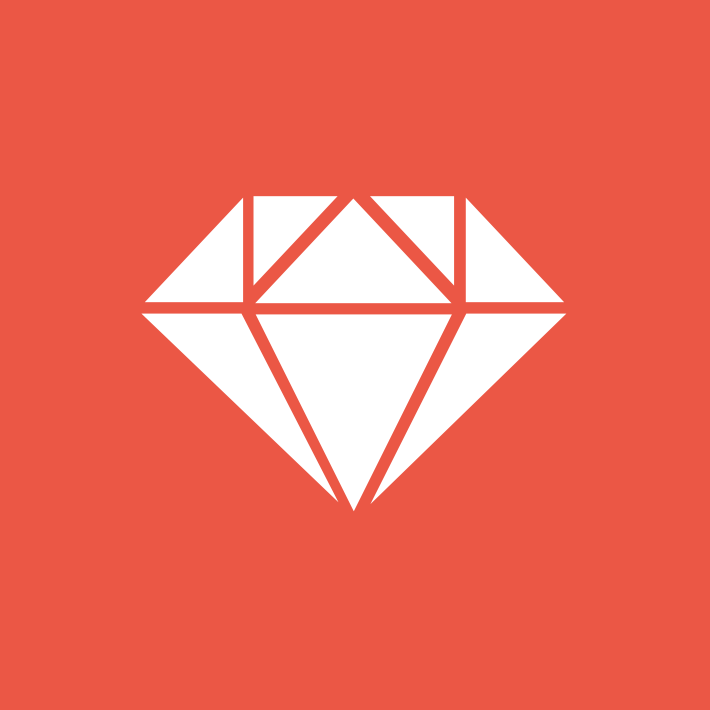
Ruby
Official HLR Lookup SDK for Ruby
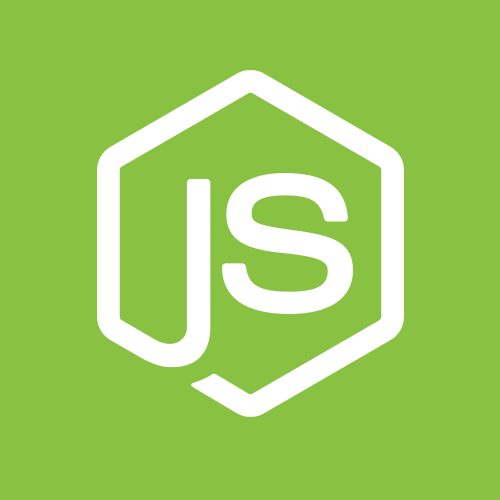
NodeJS
Official HLR Lookup SDK for NodeJS
Not a Developer?
It's easy to run HLR Lookups or Number Portability Queries even if you are not a developer. Learn more about our enterprise web client and browser-based reporting features.
Authentication
Most requests to the HLR Lookups API must be authenticated. The API endpoints are marked as public () or protected (). When accessing a protected endpoint your request must be authenticated with your API-Key using either the Basic-Auth
or Digest-Auth
method. The latter is more secure and recommended. Try the GET /auth-test
endpoint to conveniently test your authentication implementation.
API-Key and API-Secret
Visit the API Settings to obtain your API-Key and API-Secret. You should also configure your preferred authentication method and enable "IP-address whitelisting" for increased security. If you ever feel that your API-Secret has been compromised, the settings allow you to roll a new one.
Get API KeyDigest-Auth
Digest-Auth
is the recommended authentication method for accessing protected HLR Lookup API endpoints. Every request must include the following headers: X-Digest-Key
, X-Digest-Signature
, and X-Digest-Timestamp
, which are explained below.
Request
curl 'https://www.hlr-lookups.com/api/v2/auth-test' \
-H "X-Digest-Key: YOUR_API_KEY" \
-H "X-Digest-Signature: DIGEST_AUTH_SIGNATURE" \
-H "X-Digest-Timestamp: UNIX_TIMESTAMP"
Request Headers
Key | Type | Description | |
---|---|---|---|
X-Digest-Key |
string | Your HLR Lookups API Key. | mandatory |
X-Digest-Signature |
string | Unique Digest-Auth Signature (see below). | mandatory |
X-Digest-Timestamp |
integer | Current Unix timestamp (see GET /time ). |
mandatory |
The X-Digest-Signature
is generated by creating a SHA256 HMAC hash using your API-Secret as the shared secret in the message digest. The encrypted payload is composed as follows:
ENDPOINT_PATH . UNIX_TIMESTAMP . REQUEST_METHOD . REQUEST_BODY
, where .
represents string concatenation.
X-Digest-Signature Components
Component | Type | Description |
---|---|---|
ENDPOINT_PATH |
string | The path of the endpoint that is being invoked, e.g. /auth-test in lower case. |
UNIX_TIMESTAMP |
integer | Current Unix timestamp. Must be less than 30 seconds old (see GET /time ). |
REQUEST_METHOD |
string | The request method, e.g. POST or GET in upper case. |
REQUEST_BODY |
string | The request body string. Only needed in POST , PUT , or DELETE requests. Set null for GET requests. |
Code Example (PHP)
$path = '/auth-test'
$timestamp = time();
$method = 'GET';
$body = $method == 'GET' ? null : json_encode($params);
$secret = 'YOUR_API_SECRET';
$signature = hash_hmac('sha256', $path . $timestamp . $method . $body, $secret);
Code Example (Ruby)
require 'openssl'
path = '/auth-test'
timestamp = Time.now.to_i
method = 'GET'
body = method == 'GET' ? NIL : params.to_json
secret = 'YOUR_API_SECRET'
signature = OpenSSL::HMAC.hexdigest('sha256', secret, path + timestamp.to_s + method + body.to_s)
Code Example (NodeJS)
require('crypto');
let path = '/auth-test'
let timestamp = Date.now() / 1000 | 0;
let method = 'GET'
let body = method === 'GET' ? '' : JSON.stringify(params)
let secret = 'YOUR_API_SECRET'
let signature = crypto.createHmac('sha256', secret)
.update(path + timestamp + method + body)
.digest('hex');
View these examples if you're unsure how to create an HMAC SHA256 signature in your programming language.
Basic-Auth
Albeit simpler to implement, Basic-Auth
is less secure than Digest-Auth
and we recommend using the latter instead. By default HLR Lookup API docs and examples always utilize Digest-Auth
, but you can use Basic-Auth by replacing X-Digest
headers with X-Basic
, which is explained below:
Request
curl 'https://www.hlr-lookups.com/api/v2/auth-test' \
-H "X-Basic: BASIC_AUTH_HASH"
Request Headers
Key | Type | Description | |
---|---|---|---|
X-Basic |
string | SHA256 hash over YOUR_API_KEY:YOUR_API_SECRET |
mandatory |
Code Example (PHP)
$key = 'YOUR_API_KEY';
$secret = 'YOUR_API_SECRET';
$basicAuthHash = hash('sha256', $key . ':' . $secret);
IP Address Whitelisting
Use the API Settings to configure whether your API account can be accessed from any IP address or whitelisted IP addresses only. IP address whitelisting is recommended for production environments as it dramatically increases security. Withdrawal endpoints can only be accessed from whitelisted IP addresses.
SDKs (Software Development Kits)
Our Software Development Kits for PHP, Ruby, and NodeJS are here to help you quickly integrate with the HLR Lookups API without hassle.
Below is a list of SDKs currently available on our GitHub.
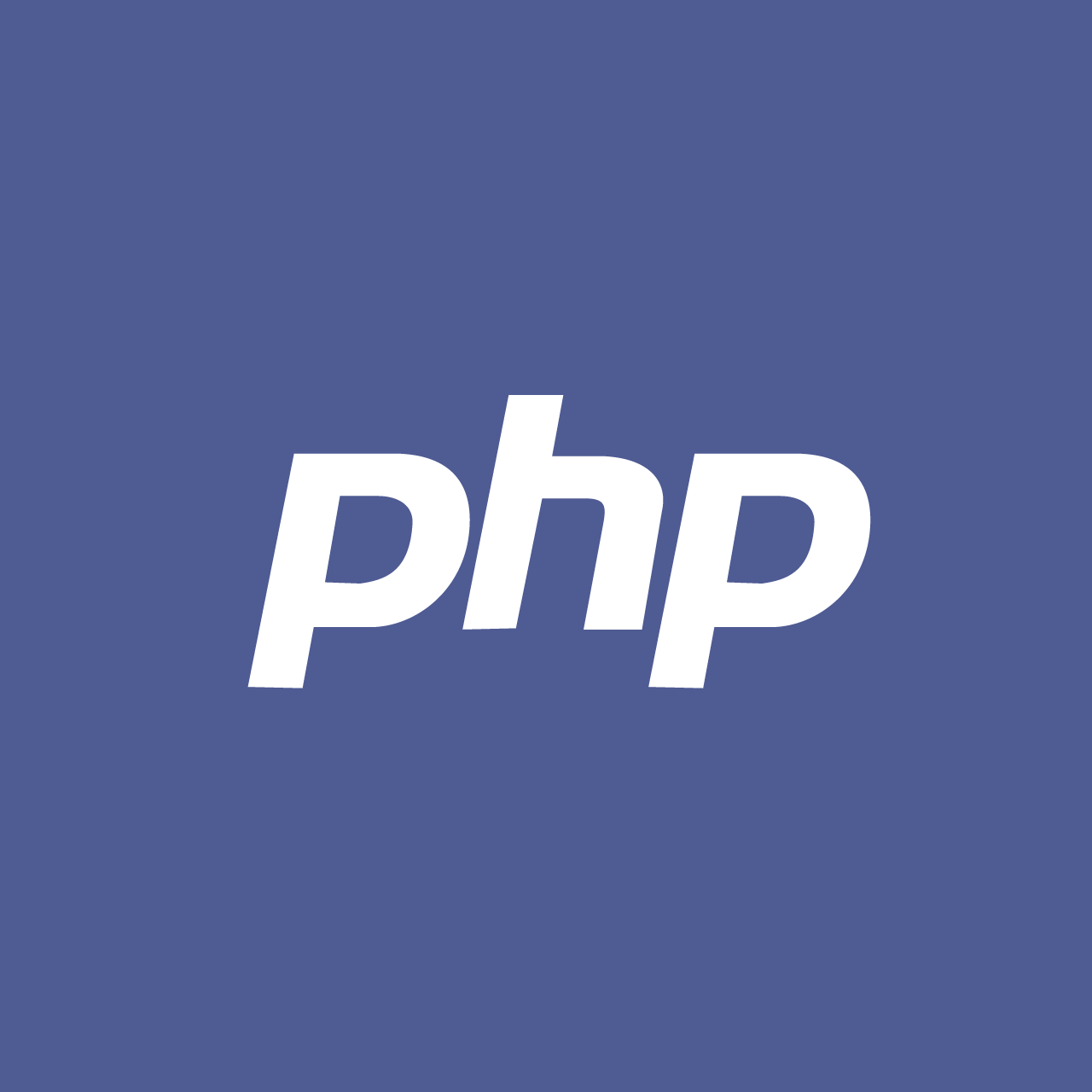
PHP
Official HLR Lookups SDK for PHP
1 include('HLRLookupClient.class.php');
2
3 $client = new HLRLookupClient(
4 'YOUR-API-KEY',
5 'YOUR-API-SECRET',
6 '/var/log/hlr-lookups.log'
7 );
8
9 $params = array('msisdn' => '+14156226819');
10 $response = $client->post('/hlr-lookup', $params);
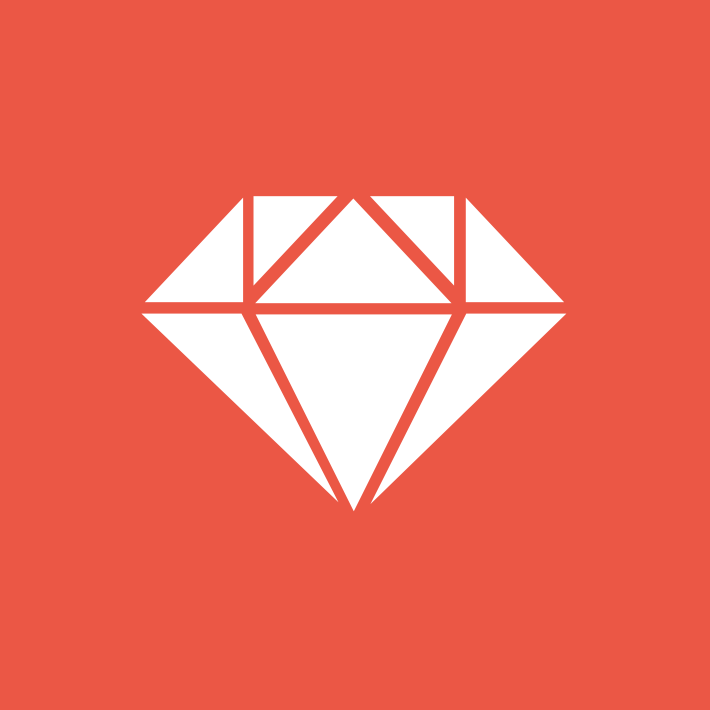
Ruby
Official HLR Lookups SDK for Ruby
1 require 'ruby_hlr_client/client'
2
3 client = HlrLookupsSDK::Client.new(
4 'YOUR-API-KEY',
5 'YOUR-API-SECRET',
6 '/var/log/hlr-lookups.log'
7 )
8
9 params = { :msisdn => '+14156226819' }
10 response = client.get('/hlr-lookup', params)
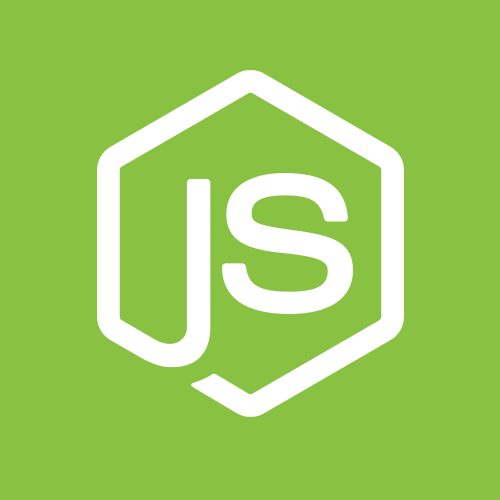
NodeJS
Official HLR Lookups SDK for NodeJS
1 require('node-hlr-client');
2
3 const client = new HlrLookupClient(
4 'YOUR-API-KEY',
5 'YOUR-API-SECRET'
6 );
7
8 const params = {msisdn:'+14156226819'};
9 const callback = function(response) {
10 console.log(response);
11 };
12 client.post('/hlr-lookup', params, callback);
POST/hlr-lookupprotected
Invokes a synchronous HLR Lookup and provides live mobile phone connectivity and portability data from network operators directly and in real-time. This endpoint is suitable for live traffic in time-critical applications and if your primary goal is to identify phone numbers that are currently reachable (connected) or absent (phone switched off) and distinguish them from invalid, unknown, or fake phone numbers. This endpoint is also suitable to extract the live portability status (MCCMNC) alongside live connectivity information.
To process large amounts of numbers that aren't time critical, please consider the asynchronous POST /hlr-lookups
endpoint (which is optimized for fast bulk processing) instead.
If portability and network information (MCCMNC) are your primary data points of interest and you don't care for connectivity information, you might want to inspect the POST /mnp-lookup
endpoint for cost-effective mobile number portability queries instead.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/hlr-lookup' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"msisdn":"+14156226819",
"route":null,
"storage":null
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
msisdn |
string | A mobile phone number (MSISDN) in international format (e.g. +14156226819 or 0014156226819). | null | mandatory |
route |
string(3) | An optional three character identifier, specifying the route for this HLR lookup. Set to null or omit to trigger automatic routing optimization. |
null | optional |
storage |
string | An optional storage name, specifying the report to which the results are appended for manual review, analytics, reporting and CSV downloads via the web interface. Set to null or omit to trigger automated reporting names grouped by months. |
null | optional |
Success Response application/json
{
"id":"f94ef092cb53",
"msisdn":"+14156226819",
"connectivity_status":"CONNECTED",
"mccmnc":"310260",
"mcc":"310",
"mnc":"260",
"imsi":"***************",
"msin":"**********",
"msc":"************",
"original_network_name":"Verizon Wireless",
"original_country_name":"United States",
"original_country_code":"US",
"original_country_prefix":"+1",
"is_ported":true,
"ported_network_name":"T-Mobile US",
"ported_country_name":"United States",
"ported_country_code":"US",
"ported_country_prefix":"+1",
"is_roaming":false,
"roaming_network_name":null,
"roaming_country_name":null,
"roaming_country_code":null,
"roaming_country_prefix":null,
"cost":"0.0100",
"timestamp":"2020-08-07 19:16:17.676+0300",
"storage":"SYNC-API-2020-08",
"route":"IP1",
"processing_status":"COMPLETED",
"error_code":null,
"data_source":"LIVE_HLR"
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
id |
string(12) | A unique identifier for this lookup. | false |
msisdn |
string | The mobile phone number inspected in this lookup request. | false |
connectivity_status |
string |
Indicates whether number type information was successfully obtained. Can be either CONNECTED , ABSENT , INVALID_MSISDN , or UNDETERMINED .
Please refer to the connectivity statuses table below for more detailed status descriptions. |
false |
mccmnc |
string(5|6) | A five or six character MCCMNC (mobile country code + mobile network code tuple) identifying the network the mobile phone number currently belongs to. | true |
mcc |
string(3) | A three character MCC (mobile country code) identifying the country the mobile phone number currently belongs to. | true |
mnc |
string(2|3) | A two or three character MNC (mobile network code) identifying the network the mobile phone number currently belongs to. | true |
imsi |
string | International Mobile Subscriber Identity (IMSI). Unique identification number associated with the SIM card. The availability of the IMSI depends on the mobile network operator. | true |
msin |
string(10) | The Mobile Subscription Identification Number (MSIN) within the mobile network operator database. The availability of the MSIN depends on the mobile network operator. | true |
msc |
string(12) | The Mobile Switching Center (MSC) currently serving the subscriber. The availability of the MSC depends on the mobile network operator. | true |
original_network_name |
string | An arbitrary string in English plain text specifying the original (native) network operator name of the inspected mobile phone number. | true |
original_country_name |
string | An arbitrary string in English plain text specifying the original country of the inspected mobile phone number. | true |
original_country_code |
string(2) | A two character ISO country code specifying the original country of the inspected mobile phone number. | true |
original_country_prefix |
string | The dialling code of the original country of the inspected mobile phone number. | true |
is_ported |
boolean | Indicates whether the number was ported from its native network to a new operator. | true |
ported_network_name |
string | Indicates the network operator to which the inspected mobile phone number was ported (if any). | true |
ported_country_name |
string | Indicates the country to which the inspected mobile phone number was ported (if any). | true |
ported_country_code |
string(2) | A two character ISO country code specifying the country to which the inspected mobile phone number was ported (if any). | true |
ported_country_prefix |
string | The dialling code of the country to which the inspected mobile phone number was ported (if any). | true |
is_roaming |
boolean | Indicates whether the number is currently roaming. The availability of roaming information depends on the mobile network operator. | true |
roaming_network_name |
string | Indicates the network in which the mobile phone is currently roaming (if any). | true |
roaming_country_name |
string | Indicates the country in which the mobile phone is currently roaming (if any). | true |
roaming_country_code |
string(2) | A two character ISO country code specifying the country in which the mobile phone is currently roaming (if any). | true |
roaming_country_prefix |
string | The dialling code of the country in which the inspected mobile phone number is currently roaming (if any). | true |
cost |
string | A decimal value as a string indicating the cost in EUR for this lookup. | true |
timestamp |
string | A W3C formatted timestamp with time zone indicating when the lookup completed. |
true |
storage |
string | Indicates the storage name to which the lookup results were appended. Refers to report name and CSV downloads via the web interface. | true |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | true |
processing_status |
string | Indicates the processing status of the HLR query. COMPLETED indicates that the query was completed. REJECTED indicates lack of connectivity to the target mobile network operator. Our platform rejected the query and no charge applies. FAILED Indicates that the HLR query was processed but some error or exception occurred. |
false |
error_code |
integer | An optional internal error code (integer) indicating further context information for customer support diagnostics. | true |
data_source |
string |
Indicates the data source used for this request. Can be either LIVE_HLR (live HLR query) or MNP_DB (static mobile number portability database). The latter can only happen on hybrid routes, which fall back to MNP when no HLR connection can be established (IP1 and IP4), or exclusive MNP routes (PTX).
Please note that results originating from MNP_DB always display a CONNECTED connectivity status as the actual reachability of the target device cannot be determined without live HLR query.
Inspect our routing options for additional information on routing. |
false |
Connectivity Statuses
Status | Description |
---|---|
CONNECTED | Indicates that the number is valid and the target handset is currently connected to the mobile network (and reachable). |
ABSENT | Indicates that the number is valid but the target handset is currently switched off or out of network reach. |
INVALID_MSISDN | Indicates that the number is not currently assigned to any subscriber on the mobile network or otherwise invalid. |
UNDETERMINED | Indicates that the connectivity status could not be determined and the connectivity status is unknown. This can be caused by invalid numbers, due to lack of connectivity to the target network operator, or other exceptions and errors. |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
POST/hlr-lookupsprotected
Invokes a series of asynchronous HLR Lookups and provides live mobile phone connectivity and portability data from network operators posted back to your server via HTTP callback. This endpoint is more suitable to process large amounts of mobile phone numbers that aren't real-time critical (such as database sanitization) than the synchronous POST /hlr-lookup
method (which should be used for live-traffic applications and real-time routing instead).
Use this endpoint for bulk processing if your primary goal is to identify phone numbers that are currently reachable (connected) or absent (phone switched off) and distinguish them from invalid, unknown or fake phone numbers. This endpoint also extracts the live portability status (MCCMNC) alongside live connectivity information.
You need to specify a webhook url on your server (to receive lookup results asynchronously) via POST /hlr-lookups-url
or your API settings as a pre-requisite to invoke this endpoint.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/hlr-lookups' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"msisdns":["+14156226819","+491788735000","+905536939360"],
"route":null,
"storage":null
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
msisdns |
array | A list of mobile phone numbers (MSISDNs) in international format (e.g. +14156226819 or 0014156226819). Accepts a maximum of 1000 numbers per request. | null | mandatory |
route |
string(3) | An optional three character identifier, specifying the route for these HLR lookups. Set to null or omit to trigger automatic routing optimization. |
null | optional |
storage |
string | An optional storage name, specifying the report to which the results are appended for manual review, analytics, reporting and CSV downloads via the web interface. Set to null or omit to trigger automated reporting names grouped by months. |
null | optional |
Success Response application/json
{
"accepted":[
{
"id":"0424928f332e",
"msisdn":"+491788735000"
}
],
"accepted_count":1,
"rejected":[
{
"id":null,
"msisdn":"+31"
}
],
"rejected_count":1,
"total_count":2,
"cost":"0.01",
"storage":"ASYNC-API-2020-08",
"route":"IP1",
"webhook_url":"https://your-server.com/endpoint"
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
accepted |
array | A list of objects with unique identifiers and MSISDNs accepted for processing. | false |
rejected |
array | A list of objects with unique identifiers and MSISDNs rejected for processing. These are typically invalid numbers. No charge applies. | false |
accepted_count |
integer | The total number of accepted MSISDNs. | false |
rejected_count |
integer | The total number of rejected MSISDNs. | false |
cost |
string | A decimal value as a string indicating the cost in EUR for these lookups. | false |
storage |
string | Indicates the storage name to which the lookup results are appended. Refers to report name and CSV downloads via the web interface. | false |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | false |
webhook_url |
string | The webhook URL specified in your API settings or via the POST /hlr-lookups-url endpoint. This is where we'll send lookup results to your server, as documented below. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
Processing Callbacks
Once submitted, our platform starts processing the given MSISDNs and posts the results back to the (previously specified) callback URL on your server. The request is sent as HTTP POST
and contains a JSON object in the HTTP request body.
The callback client expects to see a HTTP response code "200 OK" from your server. If a different response code is returned, a timeout (10 seconds) or some other error occurs, the callback client will re-attempt to send the results after a minute again. If it still encounters an error will continue do re-attempt the request after 2, 4, 8, 16, 32, 64, 128, 256, 512, and for a last time after 1024 minutes.
{
"results":[
{
"id":"3b4ac4b6ed1b",
"msisdn":"+905536939460",
"connectivity_status":"CONNECTED",
"mccmnc":"28603",
"mcc":"286",
"mnc":"03",
"imsi":"28603XXXXXXXXXX",
"msin":"XXXXXXXXXX",
"msc":"XXXXXXXXXX",
"original_network_name":"Turk Telekom (AVEA)",
"original_country_name":"Turkey",
"original_country_code":"TR",
"original_country_prefix":"+90",
"is_ported":false,
"ported_network_name":null,
"ported_country_name":null,
"ported_country_code":null,
"ported_country_prefix":null,
"is_roaming":false,
"roaming_network_name":null,
"roaming_country_name":null,
"roaming_country_code":null,
"roaming_country_prefix":null,
"cost":"0.0100",
"timestamp":"2020-08-13 00:04:38.261+0300",
"storage":"ASYNC-API-2020-08",
"route":"IP1",
"processing_status":"COMPLETED",
"error_code":null,
"data_source":"LIVE_HLR"
}
]
}
HTTP Callback JSON Object Attributes
The JSON objects contains an attribute results
with a list of HLR Lookup objects as documented below.
Name | Type | Description | Nullable |
---|---|---|---|
id |
string(12) | A unique identifier for this lookup. | false |
msisdn |
string | The mobile phone number inspected in this lookup request. | false |
connectivity_status |
string |
Indicates whether number type information was successfully obtained. Can be either CONNECTED , ABSENT , INVALID_MSISDN , or UNDETERMINED .
Please refer to the connectivity statuses table below for more detailed status descriptions. |
false |
mccmnc |
string(5|6) | A five or six character MCCMNC (mobile country code + mobile network code tuple) identifying the network the mobile phone number currently belongs to. | true |
mcc |
string(3) | A three character MCC (mobile country code) identifying the country the mobile phone number currently belongs to. | true |
mnc |
string(2|3) | A two or three character MNC (mobile network code) identifying the network the mobile phone number currently belongs to. | true |
imsi |
string | International Mobile Subscriber Identity (IMSI). Unique identification number associated with the SIM card. The availability of the IMSI depends on the mobile network operator. | true |
msin |
string(10) | The Mobile Subscription Identification Number (MSIN) within the mobile network operator database. The availability of the MSIN depends on the mobile network operator. | true |
msc |
string(12) | The Mobile Switching Center (MSC) currently serving the subscriber. The availability of the MSC depends on the mobile network operator. | true |
original_network_name |
string | An arbitrary string in English plain text specifying the original (native) network operator name of the inspected mobile phone number. | true |
original_country_name |
string | An arbitrary string in English plain text specifying the original country of the inspected mobile phone number. | true |
original_country_code |
string(2) | A two character ISO country code specifying the original country of the inspected mobile phone number. | true |
original_country_prefix |
string | The dialling code of the original country of the inspected mobile phone number. | true |
is_ported |
boolean | Indicates whether the number was ported from its native network to a new operator. | true |
ported_network_name |
string | Indicates the network operator to which the inspected mobile phone number was ported (if any). | true |
ported_country_name |
string | Indicates the country to which the inspected mobile phone number was ported (if any). | true |
ported_country_code |
string(2) | A two character ISO country code specifying the country to which the inspected mobile phone number was ported (if any). | true |
ported_country_prefix |
string | The dialling code of the country to which the inspected mobile phone number was ported (if any). | true |
is_roaming |
boolean | Indicates whether the number is currently roaming. The availability of roaming information depends on the mobile network operator. | true |
roaming_network_name |
string | Indicates the network in which the mobile phone is currently roaming (if any). | true |
roaming_country_name |
string | Indicates the country in which the mobile phone is currently roaming (if any). | true |
roaming_country_code |
string(2) | A two character ISO country code specifying the country in which the mobile phone is currently roaming (if any). | true |
roaming_country_prefix |
string | The dialling code of the country in which the inspected mobile phone number is currently roaming (if any). | true |
cost |
string | A decimal value as a string indicating the cost in EUR for this lookup. | true |
timestamp |
string | A W3C formatted timestamp with time zone indicating when the lookup completed. |
true |
storage |
string | Indicates the storage name to which the lookup results were appended. Refers to report name and CSV downloads via the web interface. | true |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | true |
processing_status |
string | Indicates the processing status of the HLR query. COMPLETED indicates that the query was completed. REJECTED indicates lack of connectivity to the target mobile network operator. Our platform rejected the query and no charge applies. FAILED Indicates that the HLR query was processed but some error or exception occurred. |
false |
error_code |
integer | An optional internal error code (integer) indicating further context information for customer support diagnostics. | true |
data_source |
string |
Indicates the data source used for this request. Can be either LIVE_HLR (live HLR query) or MNP_DB (static mobile number portability database). The latter can only happen on hybrid routes, which fall back to MNP when no HLR connection can be established (IP1 and IP4), or exclusive MNP routes (PTX).
Please note that results originating from MNP_DB always display a CONNECTED connectivity status as the actual reachability of the target device cannot be determined without live HLR query.
Inspect our routing options for additional information on routing. |
false |
Connectivity Statuses
Status | Description |
---|---|
CONNECTED | Indicates that the number is valid and the target handset is currently connected to the mobile network (and reachable). |
ABSENT | Indicates that the number is valid but the target handset is currently switched off or out of network reach. |
INVALID_MSISDN | Indicates that the number is not currently assigned to any subscriber on the mobile network or otherwise invalid. |
UNDETERMINED | Indicates that the connectivity status could not be determined and the connectivity status is unknown. This can be caused by invalid numbers, due to lack of connectivity to the target network operator, or other exceptions and errors. |
POST/hlr-lookups/urlprotected
Specifies a webhook url endpoint on your server. Our platform posts results for asynchronous HLR Lookup requests sent to the POST /hlr-lookups
endpoint to the URL specified here. Please inspect the documentation for the POST /hlr-lookups
endpoint for detailed information on how to process inbound callback requests.
The webhook endpoint url can alternatively be specified in your account settings as well.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/hlr-lookups/url' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"url":"https://user:pass@www.your-server.com:443/path/to/endpoint"
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
url |
string | A webhook URL on your server on which you want to receive callbacks for asynchronous HLR lookup requests invoked via the POST /hlr-lookups endpoint. Port and basic authentication are optional. |
null | mandatory |
Success Response application/json
{
"success":true
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
success |
boolean | Indicates the request was processed successfully. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
POST/nt-lookupprotected
Invokes a synchronous number type (NT) lookup. This endpoint is suitable if your if your primary goal is to determine whether given phone numbers belong to landline, mobile, premium rate, VoIP, pager, or other numbering plan ranges in real-time.
NT queries reliably detect the phone number type but do not indicate whether the target number is currently connected to a network and reachable. To extract live connectivity information, please use the POST /hlr-lookup
endpoint instead.
If your use case requires accurate network and portability information (MCCMNC), please use the POST /mnp-lookup
endpoint for mobile number portability queries instead.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/nt-lookup' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"number":"+14156226819",
"route":null,
"storage":null
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
number |
string | A phone number in international format (e.g. +4989702626 or 004989702626). | null | mandatory |
route |
string(3) | An optional three character identifier, specifying the route for this NT lookup. Set to null or omit to trigger automatic routing optimization. |
null | optional |
storage |
string | An optional storage name, specifying the report to which the results are appended for manual review, analytics, reporting and CSV downloads via the web interface. Set to null or omit to trigger automated reporting names grouped by months. |
null | optional |
Success Response application/json
{
"id":"2ed0788379c6",
"number":"+4989702626",
"number_type":"LANDLINE",
"query_status":"OK",
"is_valid":true,
"invalid_reason":null,
"is_possibly_ported":false,
"is_vanity_number":false,
"qualifies_for_hlr_lookup":false,
"mccmnc":null,
"mcc":null,
"mnc":null,
"original_network_name":null,
"original_country_name":"Germany",
"original_country_code":"DE",
"regions":[
"Munich"
],
"timezones":[
"Europe/Berlin"
],
"info_text":"This is a landline number.",
"cost":"0.0050",
"timestamp":"2015-12-04 10:36:41.866283+00",
"storage":"SYNC-API-NT-2015-12",
"route":"LC1"
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
id |
string(12) | A unique identifier for this lookup. | false |
number |
string | The phone number inspected in this lookup request. | false |
number_type |
string |
The detected number type. Can be either of these: LANDLINE, MOBILE , MOBILE_OR_LANDLINE , TOLL_FREE , PREMIUM_RATE , SHARED_COST , VOIP , PAGER , UAN , VOICEMAIL , UNKNOWN
Please inspect the number types table below for descriptions. |
false |
query_status |
string | Indicates whether number type information was successfully obtained. Can be either OK or FAILED . |
false |
is_valid |
boolean | Indicates whether the number is (syntactically) valid. | true |
invalid_reason |
string | Plain text in English specifying why this number is invalid (e.g. "too short", or "invalid prefix") or null if the number is valid. |
true |
is_possibly_ported |
boolean | Indicates whether the number might be ported from its original operator to a new carrier. Use MNP queries to identify actual portability. | true |
is_vanity_number |
boolean | Indicates whether this is a vanity number (i.e. using alphabetic letters). | true |
qualifies_for_hlr_lookup |
boolean | Indicates whether this number qualifies for further queries via HLR lookup. | true |
mccmnc |
string(5|6) | A five or six character MCCMNC (mobile country code + mobile network code tuple) identifying the network the mobile phone number originally belongs to. | true |
mcc |
string(3) | A three character MCC (mobile country code) identifying the country the mobile phone number originally belongs to. | true |
mnc |
string(2|3) | A two or three character MNC (mobile network code) identifying the network the mobile phone number originally belongs to. | true |
original_network_name |
string | An arbitrary string in English plain text specifying the original (native) network operator name of the inspected mobile phone number. | true |
original_country_name |
string | An arbitrary string in English plain text specifying the original country of the inspected mobile phone number. | true |
original_country_code |
string(2) | A two character ISO country code specifying the original country of the inspected mobile phone number. | true |
regions |
array | A list of human readable text in English specifying the geographic region(s) associated with this number. | true |
timezones |
array | A list of timezones associated with this number (in Olson format). | true |
info_text |
string | An arbitrary string with optional additional information about the number. | true |
cost |
string | A decimal value as a string indicating the cost in EUR for this lookup. | true |
timestamp |
string | A W3C formatted timestamp with time zone indicating when the lookup completed. |
true |
storage |
string | Indicates the storage name to which the lookup results were appended. Refers to report name and CSV downloads via the web interface. | true |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | true |
Number Types
Type | Description |
---|---|
LANDLINE | Landline phone number. |
MOBILE | Mobile phone number. Qualifies for HLR lookups to obtain additional connection status, network, portability and roaming information. |
MOBILE_OR_LANDLINE | Landline or mobile phone number. Might qualify for HLR lookup (e.g. US and Canada) |
TOLL_FREE | Toll free phone number. |
PREMIUM_RATE | Premium rate phone number with additional charges |
SHARED_COST | Shared cost phone number. Typically less expensive than premium rate phone numbers |
VOIP | Voice over IP phone number. Includes TSoIP phone numbers (Telephony Service over IP) |
PAGER | Pager phone number. Typically no voice functionality. |
UAN | Univeral Access Number (Company Number). Might be routed to specific offices but allows one number to be used for the company. |
VOICEMAIL | Voicemail phone number. |
UNKNOWN | Number type could not be determined. |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
POST/nt-lookupsprotected
Invokes a series of asynchronous number type lookups with results posted back to your server via HTTP callback. This endpoint is suitable if your if your primary goal is to determine whether given phone numbers belong to landline, mobile, premium rate, VoIP, pager, or other numbering plan ranges. This endpoint is optimized for fast processing of large amounts of numbers and should be used for bulk processing (e.g. database sanitization). For live traffic and time-critical use cases please use the synchronous POST /nt-lookup
endpoint instead.
You need to specify a webhook url on your server (to receive lookup results asynchronously) via POST /nt-lookups-url
or your API settings as a pre-requisite to invoke this endpoint.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/nt-lookups' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"numbers":["+14156226819","+4989702626"],
"route":null,
"storage":null
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
numbers |
array | A list of phone numbers in international format (e.g. +14156226819 or 004989702626). Accepts a maximum of 1000 numbers per request. | null | mandatory |
route |
string(3) | An optional three character identifier, specifying the route for these NT lookups. Set to null or omit to trigger automatic routing optimization. |
null | optional |
storage |
string | An optional storage name, specifying the report to which the results are appended for manual review, analytics, reporting and CSV downloads via the web interface. Set to null or omit to trigger automated reporting names grouped by months. |
null | optional |
Success Response application/json
{
"accepted":[
{
"id":"9f8a52cfa7d2",
"number":"+905536939460"
}
],
"accepted_count":1,
"rejected":[
{
"id":null,
"number":"+31"
}
],
"rejected_count":2,
"total_count":3,
"cost":0.005,
"storage":"ASYNC-API-NT-2020-08",
"route":"LC1",
"webhook_url":"https://your-server.com/endpoint"
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
accepted |
array | A list of objects with unique identifiers and phone numbers accepted for processing. | false |
rejected |
array | A list of objects with unique identifiers and phone numbers rejected for processing. These are typically invalid numbers. No charge applies. | false |
accepted_count |
integer | The total number of accepted phone numbers. | false |
rejected_count |
integer | The total number of rejected phone numbers. | false |
cost |
string | A decimal value as a string indicating the cost in EUR for these lookups. | false |
storage |
string | Indicates the storage name to which the lookup results are appended. Refers to report name and CSV downloads via the web interface. | false |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | false |
webhook_url |
string | The webhook URL specified in your API settings or via the POST /nt-lookups-url endpoint. This is where we'll send lookup results to your server, as documented below. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
Processing Callbacks
Once submitted, our platform starts processing the given phone numbers and posts the results back to the (previously specified) callback URL on your server. The request is sent as HTTP POST
and contains a JSON object in the HTTP request body.
{
"results":[
{
"id":"9f8a52cfa7d2",
"number":"+905536939460",
"numbertype":"MOBILE",
"state":"COMPLETED",
"isvalid":"Yes",
"invalidreason":null,
"ispossiblyported":"Yes",
"isvanitynumber":"No",
"qualifiesforhlrlookup":"Yes",
"originalcarrier":"Turk Telekom (AVEA)",
"mccmnc":"28603",
"mcc":"286",
"mnc":"03",
"countrycode":"TR",
"regions":[
"Turkey"
],
"timezones":[
"Europe\/Istanbul"
],
"infotext":"This number qualifies for HLR lookups. Determine if this subscriber number is connected, absent or invalid by running an HLR lookup. This is a mobile number and might be in roaming state. Run an HLR lookup to obtain roaming information (if available). This number is possibly ported and the carrier information might be inaccurate. To obtain portability information run an HLR lookup.",
"usercharge":"0.0050",
"inserttime":"2020-08-13 01:57:15.897+0300",
"storage":"ASYNC-API-NT-2020-08",
"route":"LC1",
"interface":"Async API"
}
]
}
HTTP Callback JSON Object Attributes
The JSON objects contains an attribute results
with a list of NT Lookup objects as documented below.
Name | Type | Description | Nullable |
---|---|---|---|
id |
string(12) | A unique identifier for this lookup. | false |
number |
string | The phone number inspected in this lookup request. | false |
number_type |
string |
The detected number type. Can be either of these: LANDLINE, MOBILE , MOBILE_OR_LANDLINE , TOLL_FREE , PREMIUM_RATE , SHARED_COST , VOIP , PAGER , UAN , VOICEMAIL , UNKNOWN
Please inspect the number types table below for descriptions. |
false |
query_status |
string | Indicates whether number type information was successfully obtained. Can be either OK or FAILED . |
false |
is_valid |
boolean | Indicates whether the number is (syntactically) valid. | true |
invalid_reason |
string | Plain text in English specifying why this number is invalid (e.g. "too short", or "invalid prefix") or null if the number is valid. |
true |
is_possibly_ported |
boolean | Indicates whether the number might be ported from its original operator to a new carrier. Use MNP queries to identify actual portability. | true |
is_vanity_number |
boolean | Indicates whether this is a vanity number (i.e. using alphabetic letters). | true |
qualifies_for_hlr_lookup |
boolean | Indicates whether this number qualifies for further queries via HLR lookup. | true |
mccmnc |
string(5|6) | A five or six character MCCMNC (mobile country code + mobile network code tuple) identifying the network the mobile phone number originally belongs to. | true |
mcc |
string(3) | A three character MCC (mobile country code) identifying the country the mobile phone number originally belongs to. | true |
mnc |
string(2|3) | A two or three character MNC (mobile network code) identifying the network the mobile phone number originally belongs to. | true |
original_network_name |
string | An arbitrary string in English plain text specifying the original (native) network operator name of the inspected mobile phone number. | true |
original_country_name |
string | An arbitrary string in English plain text specifying the original country of the inspected mobile phone number. | true |
original_country_code |
string(2) | A two character ISO country code specifying the original country of the inspected mobile phone number. | true |
regions |
array | A list of human readable text in English specifying the geographic region(s) associated with this number. | true |
timezones |
array | A list of timezones associated with this number (in Olson format). | true |
info_text |
string | An arbitrary string with optional additional information about the number. | true |
cost |
string | A decimal value as a string indicating the cost in EUR for this lookup. | true |
timestamp |
string | A W3C formatted timestamp with time zone indicating when the lookup completed. |
true |
storage |
string | Indicates the storage name to which the lookup results were appended. Refers to report name and CSV downloads via the web interface. | true |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | true |
Number Types
Type | Description |
---|---|
LANDLINE | Landline phone number. |
MOBILE | Mobile phone number. Qualifies for HLR lookups to obtain additional connection status, network, portability and roaming information. |
MOBILE_OR_LANDLINE | Landline or mobile phone number. Might qualify for HLR lookup (e.g. US and Canada) |
TOLL_FREE | Toll free phone number. |
PREMIUM_RATE | Premium rate phone number with additional charges |
SHARED_COST | Shared cost phone number. Typically less expensive than premium rate phone numbers |
VOIP | Voice over IP phone number. Includes TSoIP phone numbers (Telephony Service over IP) |
PAGER | Pager phone number. Typically no voice functionality. |
UAN | Univeral Access Number (Company Number). Might be routed to specific offices but allows one number to be used for the company. |
VOICEMAIL | Voicemail phone number. |
UNKNOWN | Number type could not be determined. |
POST/nt-lookups/urlprotected
Specifies a webhook endpoint on your server. Our platform posts results for asynchronous HLR Lookup requests sent to the POST /nt-lookups
endpoint to the URL specified here. Please inspect the documentation for the POST /nt-lookups
endpoint for detailed information on how to process inbound callback requests.
The webhook endpoint can alternatively be specified in your account settings as well.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/nt-lookups/url' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"url":"https://user:pass@www.your-server.com:443/path/to/endpoint"
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
url |
string | A webhook URL on your server on which you want to receive callbacks for asynchronous NT lookup requests invoked via the POST /nt-lookups endpoint. Port and basic authentication are optional. |
null | mandatory |
Success Response application/json
{
"success":true
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
success |
boolean | Indicates the request was processed successfully. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
POST/mnp-lookupprotected
Invokes a synchronous mobile number portability (MNP) lookup. This endpoint is suitable if your if your primary goal is to extract the current MCCMNC of a given mobile phone number and pinpoint the original and current networks in real-time.
MNP queries reliably determine portability and network information but do not indicate whether the target mobile phone is currently connected to a network and reachable. To extract live connectivity information, please use the POST /hlr-lookup
endpoint instead.
Request
curl -X POST 'https://www.hlr-lookups.com/api/v2/mnp-lookup' \
-d "@payload.json"
Where payload.json is a JSON object:
Payload
{
"msisdn":"+14156226819",
"route":null,
"storage":null
}
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
msisdn |
string | A mobile phone number (MSISDN) in international format (e.g. +14156226819 or 0014156226819). | null | mandatory |
route |
string(3) | An optional three character identifier, specifying the route for this MNP lookup. Set to null or omit to trigger automatic routing optimization. |
null | optional |
storage |
string | An optional storage name, specifying the report to which the results are appended for manual review, analytics, reporting and CSV downloads via the web interface. Set to null or omit to trigger automated reporting names grouped by months. |
null | optional |
Success Response application/json
{
"id":"e428acb1c0ae",
"msisdn":"+14156226819",
"query_status":"OK",
"mccmnc":"310260",
"mcc":"310",
"mnc":"260",
"is_ported":true,
"original_network_name":"Verizon Wireless:6006 - SVR/2",
"original_country_name":"United States",
"original_country_code":"US",
"original_country_prefix":"+1415",
"ported_network_name":"T-Mobile US:6529 - SVR/2",
"ported_country_name":"United States",
"ported_country_code":"US",
"ported_country_prefix":"+1",
"extra":"LRN:4154250000",
"cost":"0.0050",
"timestamp":"2020-08-05 21:21:33.490+0300",
"storage":"WEB-CLIENT-SOLO-MNP-2020-08",
"route":"PTX",
"error_code":null
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
id |
string(12) | A unique identifier for this lookup. | false |
msisdn |
string | The mobile phone number inspected in this lookup request. | false |
query_status |
string | Indicates whether portability and network information were successfully obtained. Can be either OK or FAILED . |
false |
mccmnc |
string(5|6) | A five or six character MCCMNC (mobile country code + mobile network code tuple) identifying the network the mobile phone number currently belongs to. | true |
mcc |
string(3) | A three character MCC (mobile country code) identifying the country the mobile phone number currently belongs to. | true |
mnc |
string(2|3) | A two or three character MNC (mobile network code) identifying the network the mobile phone number currently belongs to. | true |
is_ported |
boolean | Indicates whether the number was ported from its native network to a new operator. | true |
original_network_name |
string | An arbitrary string in English plain text specifying the original (native) network operator name of the inspected mobile phone number. | true |
original_country_name |
string | An arbitrary string in English plain text specifying the original country of the inspected mobile phone number. | true |
original_country_code |
string(2) | A two character ISO country code specifying the original country of the inspected mobile phone number. | true |
original_country_prefix |
string | The dialling code of the original country of the inspected mobile phone number. | true |
ported_network_name |
string | Indicates the network operator to which the inspected mobile phone number was ported (if any). | true |
ported_country_name |
string | Indicates the country to which the inspected mobile phone number was ported (if any). | true |
ported_country_code |
string(2) | A two character ISO country code specifying the country to which the inspected mobile phone number was ported (if any). | true |
ported_country_prefix |
string | The dialling code of the country to which the inspected mobile phone number was ported (if any). | true |
extra |
string | An arbitrary string with optional additional information about the number. | true |
cost |
string | A decimal value as a string indicating the cost in EUR for this lookup. | true |
timestamp |
string | A W3C formatted timestamp with time zone indicating when the lookup completed. |
true |
storage |
string | Indicates the storage name to which the lookup results were appended. Refers to report name and CSV downloads via the web interface. | true |
route |
string(3) | A three character identifier indicating the route used for this lookup request. | true |
error_code |
integer | An optional internal error code (integer) indicating further context information for customer support diagnostics. | true |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
GET/mnp-coverageprotected
This endpoint returns a list of mobile network operators (along with their corresponding MCCMNC identifier) currently available for mobile number portability lookups.
Request
curl 'https://www.hlr-lookups.com/api/v2/mnp-coverage?countrycode=XX'
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
countrycode |
string(2) | An optional two-letter ISO country code to filter the MCCMNC results by. | null | optional |
Success Response application/json
{
"items":[
{
"country_name":"Germany",
"country_code":"DE",
"mccmnc":"26201",
"mcc":"262",
"mnc":"01 ",
"brand":"Telekom",
"operator":"Telekom Deutschland GmbH"
},
{
"country_name":"Germany",
"country_code":"DE",
"mccmnc":"26202",
"mcc":"262",
"mnc":"02 ",
"brand":"Vodafone",
"operator":"Vodafone D2 GmbH"
}
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
.items[] |
array | A list of mobile network operators. | false |
.country_name |
string | A country name in English plain text. | false |
.country_code |
string(2) | A two-letter ISO country code. | false |
.mccmnc |
string(5|6) | A five or six character string containing the mobile network operator's MCCMNC. | false |
.mcc |
string(3) | A three character string containing the country's MCC (mobile country code). | false |
.mnc |
string(2|3) | A two or three character string containing the mobile network operator's MNC (mobile network code). | false |
.brand |
string | The brand under which this network is known to consumers and end users. | true |
.operator |
string | The mobile network operator's name and legal entity. | true |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
GET/mccmncsprotected
This endpoint returns a list of mobile network operators along with their corresponding MCCMNC identifier and additional context information. Based on this GitHub repository.
Request
curl 'https://www.hlr-lookups.com/api/v2/mccmncs?countrycode=XX'
Request Params
Key | Type | Description | Default | Mandatory |
---|---|---|---|---|
countrycode |
string(2) | An optional two-letter ISO country code to filter the MCCMNC results by. | null | optional |
Success Response application/json
{
"items":[
{
"country_name":"Germany",
"country_code":"DE",
"mccmnc":"26201",
"mcc":"262",
"mnc":"01 ",
"brand":"Telekom",
"operator":"Telekom Deutschland GmbH",
"bands":"GSM 900 / GSM 1800 / UMTS 2100 / LTE 800 / LTE 900 / LTE 1500 / LTE 1800 / LTE 2600 / 5G 3500",
"notes":"Formerly D1 - DeTeMobil, D1-Telekom, T-D1, T-Mobile"
},
{
"country_name":"Germany",
"country_code":"DE",
"mccmnc":"26202",
"mcc":"262",
"mnc":"02 ",
"brand":"Vodafone",
"operator":"Vodafone D2 GmbH",
"bands":"GSM 900 / GSM 1800 / UMTS 2100 / LTE 700 / LTE 800 / LTE 900 / LTE 1800 / LTE 2600 / 5G 3500",
"notes":"Former D2 Mannesmann"
}
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
.items[] |
array | A list of mobile network operators. | false |
.country_name |
string | A country name in English plain text. | false |
.country_code |
string(2) | A two-letter ISO country code. | false |
.mccmnc |
string(5|6) | A five or six character string containing the mobile network operator's MCCMNC. | false |
.mcc |
string(3) | A three character string containing the country's MCC (mobile country code). | false |
.mnc |
string(2|3) | A two or three character string containing the mobile network operator's MNC (mobile network code). | false |
.brand |
string | The brand under which this network is known to consumers and end users. | true |
.operator |
string | The mobile network operator's name and legal entity. | true |
.bands |
string | A list of bands on which the network operates. | true |
.notes |
string | An optional text containing additional meta information for humans. | true |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
GET/balanceprotected
This endpoint returns your current account balance. It can help you automate additional logic alongside the automated low credit notification emails configurable on your payments page.
Request
curl 'https://www.hlr-lookups.com/api/v2/balance'
Success Response application/json
{
"balance":"1002.90"
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
balance |
string | Your current account balance in EUR. A decimal value of type string. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
GET/pingpublic
Sends a ping request to the API. This endpoint is a good starting place in trying to initially connect to the HLR Lookups API.
Request
curl 'https://www.hlr-lookups.com/api/v2/ping'
Success Response application/json
{
"success":true
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
success |
boolean | Indicates the request was processed successfully. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
GET/timepublic
Returns a Unix timestamp with the current HLR Lookups server time. Use this endpoint when generating the Digest-Auth
signature for authentication if you feel time the between your server and the HLR Lookups server is askew.
Request
curl 'https://www.hlr-lookups.com/api/v2/time'
Success Response application/json
{
"time":1525898643
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
time |
integer | Unix timestamp with HLR Lookups server time. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
GET/auth-testprotected
A good starting place to test your Basic-Auth
or (recommended) Digest-Auth
implementation. The below example demonstrates a Digest-Auth
request. This endpoint accepts Basic-Auth
but it is discouraged for production environments. To use Basic-Auth
, replace the X-Digest
headers with the X-Basic
one (see Authentication).
Request
curl 'https://www.hlr-lookups.com/api/v2/auth-test' \
-H "X-Digest-Key: YOUR_API_KEY" \
-H "X-Digest-Signature: DIGEST_AUTH_SIGNATURE" \
-H "X-Digest-Timestamp: UNIX_TIMESTAMP"
Request Headers
Key | Type | Description |
---|---|---|
X-Digest-Key |
string | Your HLR Lookups API Key |
X-Digest-Signature |
string | Unique Digest-Auth signature (see authentication) |
X-Digest-Timestamp |
integer | Current Unix timestamp (also see GET /time ). |
Success Response application/json
{
"success":true
}
Success Response Attributes
Name | Type | Description | Nullable |
---|---|---|---|
success |
boolean | Indicates the request was processed successfully. | false |
Error Response application/json
{
"errors":[
"Service unavailable."
]
}
Error Response Params
Name | Type | Description | Nullable |
---|---|---|---|
errors[] |
string[ ] | A list of strings explaining the error. | false |
Legacy API Docs
If your system implemented HLR Lookups between 2015 and early 2020 you are using our legacy API. Please refer to our legacy API documentation in that case.
Please note that the legacy API is deprecated and is scheduled for retirement in the future. We highly recommend to upgrade to the latest version at your earliest convenience.
Legacy API Docs